Building a Dashboard with Next JS and Tailwind
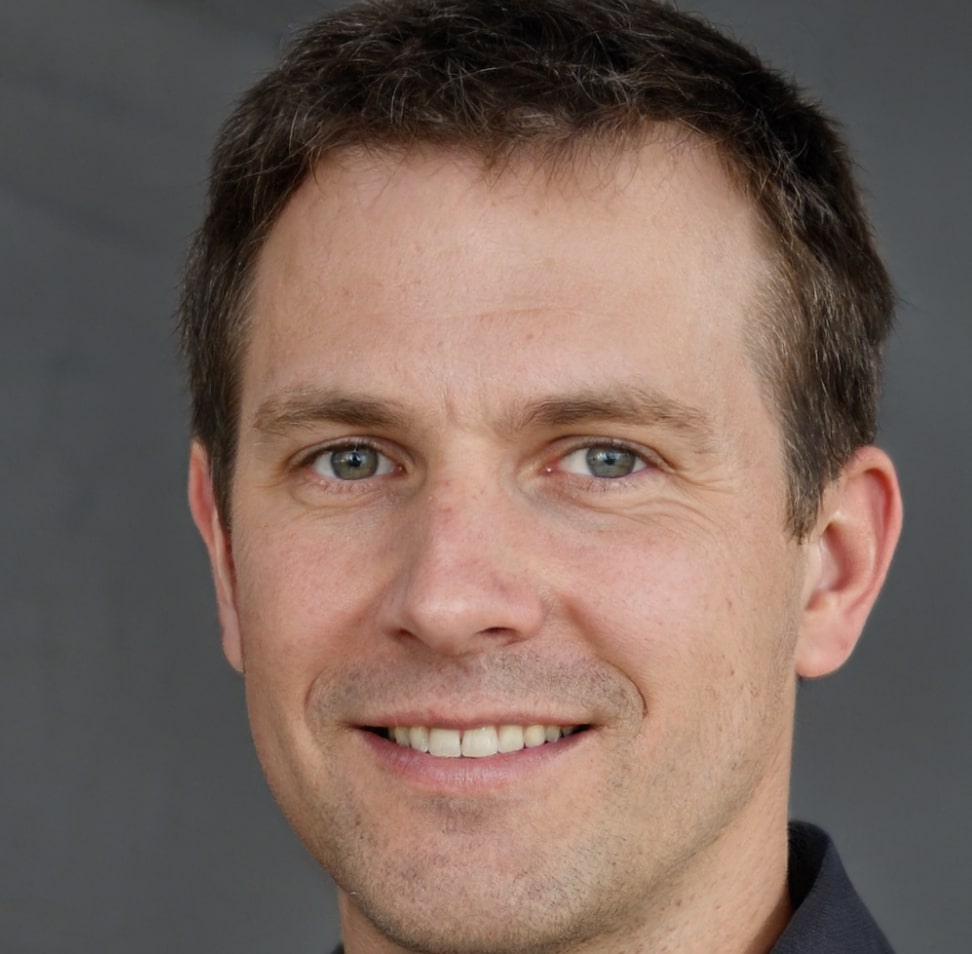
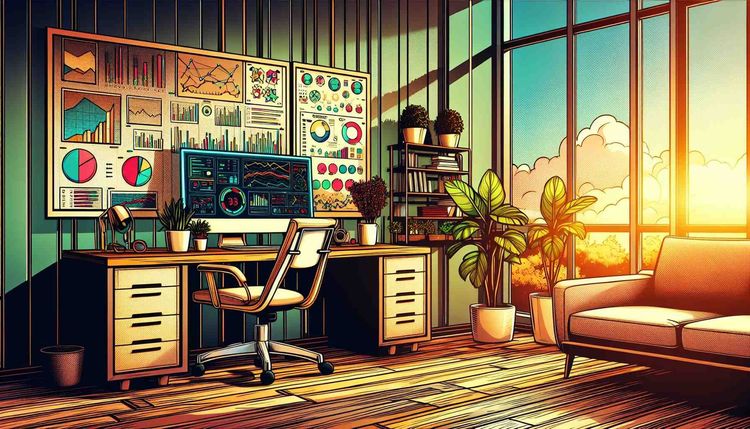
Table of Contents
- Why Next.js and Tailwind for Dashboards?
- Getting Started
- Creating the Dashboard Layout
- Implementing Responsive Design
- Adding Data Visualization
- Performance Optimization
- Advanced Features
- Wrap-up
- FAQ
Why Next.js and Tailwind for Dashboards?
Dashboards have become the nerve center of modern web applications, providing users with at-a-glance insights and controls. When it comes to building these crucial interfaces, the combination of Next.js and Tailwind CSS has emerged as a powerhouse duo. Next.js, with its server-side rendering capabilities, offers lightning-fast load times, while Tailwind's utility-first approach allows for rapid UI development without sacrificing customization.
Let's dive into why this tech stack is gaining traction among developers for dashboard creation:
- Next.js provides optimized performance out of the box
- Tailwind CSS enables quick styling with minimal CSS
- Both technologies have strong community support and extensive documentation
- The combination allows for highly responsive and interactive dashboards
Getting Started
To begin building your dashboard, you'll need to set up a new Next.js project and integrate Tailwind CSS. Here's a quick rundown of the initial steps:
- Create a new Next.js project using create-next-app
- Install Tailwind CSS and its dependencies
- Configure Tailwind by creating a tailwind.config.js file
- Set up your global styles in globals.css
Once you have your environment set up, you're ready to start crafting your dashboard components. The key to a successful dashboard is a well-thought-out component structure. This allows for easier maintenance and scalability as your project grows.
Creating the Dashboard Layout
The foundation of any good dashboard is its layout. With Next.js and Tailwind, you can create a flexible and maintainable structure. Here's a basic layout you might consider:
- Header component with navigation and user info
- Sidebar for main navigation categories
- Main content area for widgets and data displays
- Footer for additional links or information
Using Tailwind's grid and flexbox utilities, you can easily create responsive layouts that adapt to different screen sizes. Here's an example of how you might structure your main dashboard component:
<div className="flex h-screen bg-gray-100">
<Sidebar />
<div className="flex flex-col flex-1">
<Header />
<main className="flex-1 overflow-y-auto p-5">
{/* Dashboard content */}
</main>
<Footer />
</div>
</div>
This structure provides a solid foundation for building out your dashboard's various sections and components.
Implementing Responsive Design
Responsiveness is crucial for modern dashboards. Users expect to access their data on various devices, from desktop monitors to smartphones. Tailwind makes responsive design a breeze with its mobile-first approach and responsive utility classes.
Here are some tips for creating a responsive dashboard:
- Use Tailwind's responsive prefixes (sm:, md:, lg:, xl:) to adjust layouts for different breakpoints
- Implement a collapsible sidebar for mobile views
- Utilize Flexbox and Grid for flexible content arrangement
- Adjust font sizes and spacing for readability on smaller screens
Responsive design isn't just about fitting content on smaller screens; it's about optimizing the user experience across all devices. Consider how users interact with your dashboard on different devices and tailor the interface accordingly.
Adding Data Visualization
A dashboard without data visualization is like a car without wheels. Integrating charts, graphs, and other visual representations of data is essential for providing users with meaningful insights at a glance. While Next.js and Tailwind don't provide built-in charting capabilities, they integrate well with popular libraries like Chart.js or D3.js.
Here's a simple example of how you might integrate a Chart.js component into your Next.js dashboard:
import { Line } from 'react-chartjs-2';
const LineChart = ({ data }) => {
return (
<div className="bg-white p-4 rounded-lg shadow">
<Line data={data} options={options} />
</div>
);
};
When selecting a data visualization library, consider factors such as:
- Performance and bundle size impact
- Customization options
- Ease of integration with Next.js
- Community support and documentation
Performance Optimization
Performance is paramount when it comes to dashboards, especially those handling large amounts of data. Next.js provides several features out of the box to help optimize your dashboard's performance:
- Automatic code splitting for faster page loads
- Image optimization with the Next.js Image component
- API routes for serverless function support
Additionally, you can leverage Tailwind's purge option to remove unused styles in production, significantly reducing your CSS bundle size. Here's a comparison of bundle sizes before and after optimization:
Metric | Before Optimization | After Optimization |
---|---|---|
JS Bundle Size | 250KB | 180KB |
CSS Bundle Size | 100KB | 10KB |
First Contentful Paint | 1.5s | 0.8s |
These optimizations can lead to significant improvements in load times and overall user experience.
Advanced Features
Once you have the basics of your dashboard in place, you can start adding more advanced features to enhance functionality and user experience. Some popular advanced features include:
- Real-time data updates using WebSockets or Server-Sent Events
- Drag-and-drop interfaces for customizable layouts
- Advanced filtering and search capabilities
- Export options for data and reports
Implementing these features can significantly increase the complexity of your dashboard. This is where using a pre-built solution can save you time and effort. For instance, BoilerplateHub.com offers dashboard boilerplates that come with many of these advanced features already implemented, allowing you to focus on customizing the dashboard for your specific needs.
Wrap-up
Building a dashboard with Next.js and Tailwind offers a powerful combination of performance, flexibility, and ease of development. By leveraging the strengths of both technologies, you can create responsive, data-rich interfaces that provide value to your users.
Remember, the key to a successful dashboard lies in understanding your users' needs and presenting data in a clear, actionable format. While building from scratch gives you complete control, it can also be time-consuming. That's why many developers turn to pre-built solutions like those offered on BoilerplateHub.com to jumpstart their dashboard projects.
Whether you choose to build from the ground up or start with a boilerplate, the most important thing is to create a dashboard that serves your users' needs effectively.
FAQ
Q: How long does it typically take to build a custom dashboard with Next.js and Tailwind?
A: The time can vary greatly depending on the complexity of the dashboard and your familiarity with the technologies. A basic dashboard might take a few days, while a complex, data-heavy dashboard could take several weeks or more.
Q: Can I use TypeScript with Next.js for my dashboard project?
A: Yes, Next.js has excellent TypeScript support. Many developers prefer using TypeScript for larger projects due to its type safety and improved developer experience.
Q: How can I ensure my dashboard is accessible to all users?
A: Focus on semantic HTML, proper color contrast, keyboard navigation, and ARIA attributes. Tailwind provides utilities to help with many accessibility concerns, but you'll need to be intentional about implementing them.
Q: Are there any performance concerns when building large dashboards with Next.js?
A: While Next.js is generally very performant, large dashboards with many components and data points can become slow if not optimized properly. Use techniques like code splitting, lazy loading, and efficient state management to maintain performance.